Python Keywords: with
Python Keywords: with
建议点击 查看原文 查看最新内容。
原文链接: https://typonotes.com/posts/2024/11/22/python-keywords-with/
with
是 python 中的一个关键字。 一种更简单的方式实现 try...catch
。
例如, 打开文件后获得文件句柄f, 无论执行是否正常都需要关闭句柄。
|
|
但是使用 with
关键字, 就可以简单的写成如下
|
|
with...as
的类实现: ContextManager
with 执行的对象需要实现两个 内置方法
__enter__()
__exit__()
只要有这两个方法, 就算实现了 ContextManager
, 就可以使用 with 捕获。
|
|
这个有点像 golang 中的接口
|
|
with...as
的函数实现
除了类之外, 函数也可以通过 contextlib
的方式实现 Context Manager
注意:
contextlib
是标准库
|
|
注意: 这里 只能 使用
yield
返回句柄。
参考文档
- 原文链接:https://typonotes.com/posts/2024/11/22/python-keywords-with/
- 本文为原创文章,转载注明出处。
- 欢迎 扫码关注公众号
Go与云原生
或 订阅网站 https://typonotes.com/ 。 - 第一时间看后续精彩文章。觉得好的话,请猛击文章右下角「在看」,感谢支持。
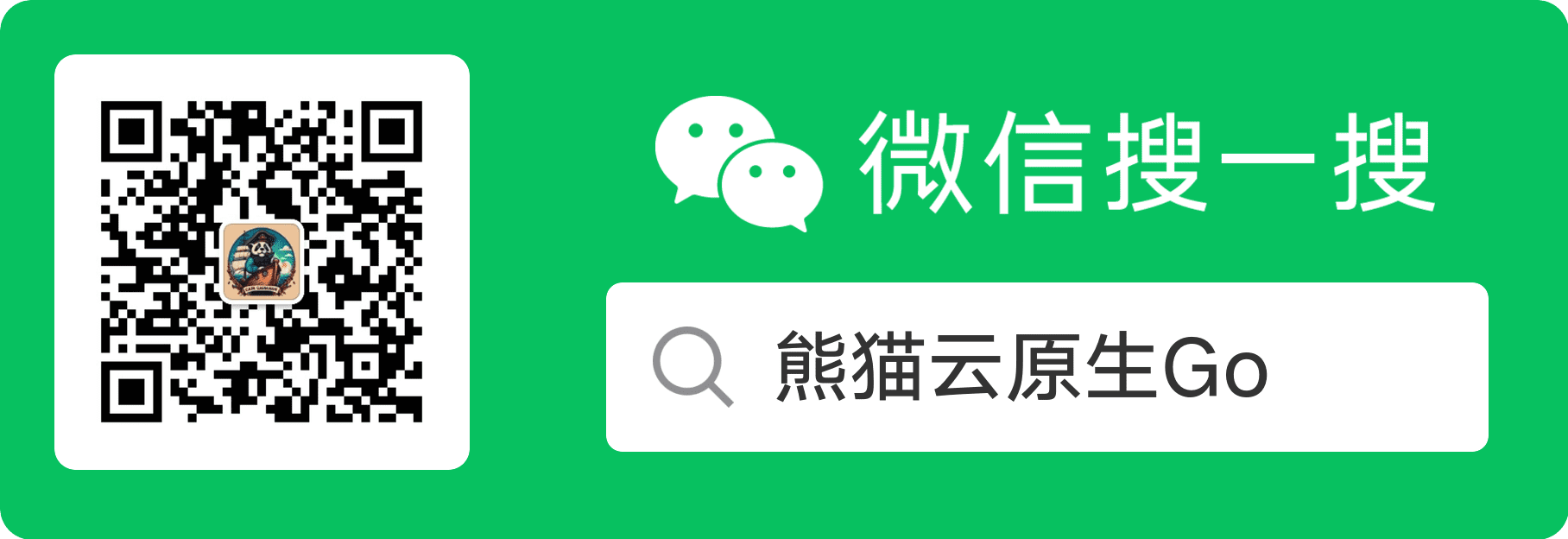